Creating Internal Processing (MS)
Create an execution file for all actual internal processing such as measurement.
Implementing Internal Processing
FJ uses separate files to write the processing of each event, allowing each file to be edited for each required event. The tutorial describes how to edit the following seven files:
- ItemDefs.h: Defines the data structure
- ItemInit.cpp: Sets the attribute for the processing item to be created
- AssignProc.cpp: Writes the processing performed when a processing item is added to the flow
- FigureSetup.cpp: Writes the processing performed when figure data is updated
- MeasureProc.cpp: Writes the processing for executing measurement
- MeasureDisp.cpp: Writes the processing for displaying measurement results
- UnitData.cpp: Writes the processing that gets/sets data from outside the processing item's MS
Implementing ItemDefs
Define the data structure. Define the settings data required to execute the processing in the SETUPDATA structure, and the measurement data required to store the measurement results in the MEASUREDATA structure.
Sample Program
Implementing ItemInit
Set the attribute information for the processing item to be created. Set the processing item information in the predetermined items of CLASSNAME(). Since ItemDefs.h is created with input data in the FJ processing item creation wizard, it doesn't need to be edited in the sample program.
Sample Program
|
Since the sample program is for measurement processing, its attribute is set to "general measurement".
|
|
The sample program is designed with figure data 0 registering the model data, and with figure data 1 setting the measurement region.
|
|
modelDataCount specifies the number of memory areas to register model data of a given size. It is set to "1" because the search processing handled in the sample program needs to retain the image data to be searched for.
|
Implementing AssignProc Processing
In AssignProc(), write the processing to be executed when a processing item is added to the flow. Mainly, data initialization is performed.
Sample Program
|
Obtain a pointer to the area of the settings data retained by the MS item. The structure of the settings data is SETUPDATA as defined in ItemDefs.h.
|
|
Set the format information of the figure data. With SetFigureType(), set the figure format for the specified figure number.
The sample program is designed so that figure data 0 can use "rectangle"/"ellipse", and figure data 1 can use "rectangle".
|
|
Set an initial value for the figure data. The initial value is stored in the figure data structure, and is set with AddFigureData().
|

Note
- The figures available for the UI figure setting control are determined according to the format information set in (2).
Implementing FigureUpdate Processing
In FigureUpdate(), write the processing to be executed when the figure data is updated. Figure data update processing is executed when a figure setting control/API is executed in the UI.
Described below is the model registration processing to be executed at figure change/registration/deletion.
Sample Program
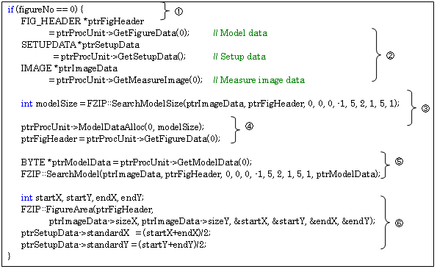
|
The sample program defines data for two figures, with figure data number 0 being model data, and figure data number 1 being measurement region data. [Note 1]
The model registration process is to run only when the figure data number 0 requiring model registration is received.
|
|
Get the data required for the model data registration processing. Use GetFigureData() to get the changed figure data, GetSetupData() to get the settings data, and GetMeasureImage() to get the image data to be measured.
|
|
Calculate the model data size.
Use SearchModelSize() to get the model size. [Note 2]
|
|
Secure the model data storage area. Using the API in FZW, allocate the storage area for the model data according to the following procedure: [Note 3]
- Use ModelDataAlloc() to allocate the area of the model size.
- Use GetFigureData() to reallocate the figure data again because the figure data address is changed after the area is secured with ModelDataAlloc().
|
|
Store the model data in the allocated area.
- Use GetModelData() to get the address of the allocated area.
- Use SearchModel() to store the model data in the allocated area.[Note 2]
|
|
Set the data determined at model registration.
Since the "reference position" of the model figure's coordinates is determined at model registration in search processing, the model data needs to be stored in the setting data. The reference position data set here is used at measurement execution.
|
[Note 1]: The MS-defined figure number for model data must match the figure number set in the UI figure setting control property "DataIdentNum_Figure" explained in "Create the Setting Window User Interface (UI)".
[Note 2]: The method for registering model data differs depending on the measurement library API used; in this sample program, model registration for search processing is written. For the measurement library, see "
Measurement Library Reference".
[Note 3]: For memory allocation/freeing, use the API provided by FJ.
Implementing MeasureProc Processing
In MeasureProc(), write the processing when executing the measurement. The processing procedure differs depending on the measurement library used.
Sample Program
|
Get the data required for the measurement processing.
Use GetSetupData() to get the settings data, GetModelData() to get the model data registered in FigureUpdate.cpp, GetMeasureImage() to get the image data to be measured, and GetFigureData(1) to get the figure data indicating the measurement region.
|
|
Get the data to store the measurement results.
Use GetMeasureData() to get the data for storing the measurement results.
|
|
Execute the measurement processing.
In the sample program the search for the region which is similar to the model registered via Search() is executed.
|
|
Execute determination processing after the measurement is completed.
In the sample program it is considered an error if Search 0 returns an error. The judgement result set in setUnitJudge() is the judgement result of the unit.
|

Note
Implementing MeasureDisp Processing
Write the processing for displaying measurement results. The display processing is divided into the following three functions:
- MeasureDispI(): Writes the processing that displays the image in the Measurement window
- MeasureDispG(): Writes the processing that displays the graphics
- MeasureDispT(): Writes the processing that displays detailed results as text
Sample Program: MeasureDispI()
No change is made to the program created in the model form. Use GetMeasureImage(0) to get the image to be measured, and ImageDisp() to display it in the Measurement window.
Sample Program: MeasureDispG()
|
Display the measurement region. Use GetFigureData(1) to get the figure data in the measurement region, and DrawFigure() to display the figure data.
|
|
Display the model figure. Pass the data for the figure size, reference position, and measurement position to DrawSearchFigure()to display the detection position.
|
Sample Program: MeasureDispT()
Get the measurement result data, and use DrawText() to display the content in text.
Implementing UnitData Processing
Write the processing that gets/sets data from outside the processing item MS.
For example, write the process to get/set a setting data to be displayed in the Setting window (UI) when you open/close the Setting window.
The getting/setting process is divided into the following two functions:
- SetUnitData(): Writes the processing that sets data when a settings data/measurement data is specified from outside the item
- GetUnitData(): Writes the processing that gets data when a settings data/measurement data is specified from outside the item
- Setting Data
In SetUnitData(), write the processing that sets data from outside to a processing item MS. SetUnitData() has the following two interfaces:
- int SetUnitData(ProcUnit *ptrProcUnit, TCHAR *dataIdent, ANYTYPE *data)
Specify a settings data item with the string data "dataIdent".
- int SetUnitData(ProcUnit *ptrProcUnit, int dataNo, ANYTYPE *data)
Specify a settings data item with the integer data "dataNo".
In the sample program, the settings data item is specified as "string data", therefore, the processing is written in "int SetUnitData(ProcUnit *ptrProcUnit, TCHAR *dataIdent, ANYTYPE *data)".
Sample Program: SetUnitData()
|
Obtain a pointer to the settings data area retained by the MS item.
The structure of the settings data is SETUPDATA as defined in ItemDefs.h.
|
|
When "candidateLevel" is specified, set the data passed from outside the item in the settings data "candidateLevel".
|
- Getting Data
In GetUnitData(), write the processing that gets data retained by a processing item MS from outside. GetUnitData() has the following two interfaces:
- int GetUnitData(ProcUnit *ptrProcUnit, TCHAR *dataIdent, ANYTYPE *data)
Specify a settings data item with the string data "dataIdent".
- int GetUnitData(ProcUnit *ptrProcUnit, int dataNo, ANYTYPE *data)
Specify a settings data item with the integer data "dataNo".
In the sample program, the settings data item is specified as "string data", so the processing is written in "int GetUnitData(ProcUnit *ptrProcUnit, TCHAR *dataIdent, ANYTYPE *data)".
|
Obtain pointers to the settings data area and the measurement data area retained by the MS item.
The data structures are SETUPDATA and MEASUREDATA as defined in InitDef.h respectively.
|
|
When "candidateLevel" is specified, pass the data set in "candidateLevel" outside the item.
|
Building a Program
Execute [Build] - [Build solution] from the menu, and an execution file is created. If an error occurs during building, correct the error.
The created files are for "Release" and "Debug", which are toggled by "Configure solution" in Visual Studio. For debugging, use the file created for "Debug".